mirror of
https://github.com/yggdrasil-network/yggdrasil-android.git
synced 2025-07-14 20:05:08 +03:00
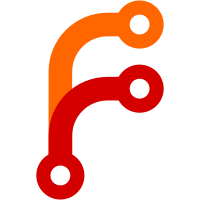
* Implemented an option to reset keys (and IP). * Implemented an option to set own keys (and IP). * Incremented app version.
97 lines
No EOL
2.8 KiB
Kotlin
97 lines
No EOL
2.8 KiB
Kotlin
package eu.neilalexander.yggdrasil
|
|
|
|
import android.content.Context
|
|
import mobile.Mobile
|
|
import org.json.JSONArray
|
|
import org.json.JSONObject
|
|
import java.io.File
|
|
|
|
object ConfigurationProxy {
|
|
private lateinit var json: JSONObject
|
|
private lateinit var file: File
|
|
|
|
operator fun invoke(applicationContext: Context): ConfigurationProxy {
|
|
file = File(applicationContext.filesDir, "yggdrasil.conf")
|
|
if (!file.exists()) {
|
|
val conf = Mobile.generateConfigJSON()
|
|
if (file.createNewFile()) {
|
|
file.writeBytes(conf)
|
|
}
|
|
}
|
|
fix()
|
|
return this
|
|
}
|
|
|
|
fun resetJSON() {
|
|
val conf = Mobile.generateConfigJSON()
|
|
file.writeBytes(conf)
|
|
fix()
|
|
}
|
|
|
|
fun resetKeys() {
|
|
val newJson = JSONObject(String(Mobile.generateConfigJSON()))
|
|
updateJSON { json ->
|
|
json.put("PrivateKey", newJson.getString("PrivateKey"))
|
|
json.put("PublicKey", newJson.getString("PublicKey"))
|
|
}
|
|
}
|
|
|
|
fun setKeys(privateKey: String, publicKey: String) {
|
|
updateJSON { json ->
|
|
json.put("PrivateKey", privateKey)
|
|
json.put("PublicKey", publicKey)
|
|
}
|
|
}
|
|
|
|
fun updateJSON(fn: (JSONObject) -> Unit) {
|
|
json = JSONObject(file.readText(Charsets.UTF_8))
|
|
fn(json)
|
|
val str = json.toString()
|
|
file.writeText(str, Charsets.UTF_8)
|
|
}
|
|
|
|
private fun fix() {
|
|
updateJSON { json ->
|
|
json.put("AdminListen", "none")
|
|
json.put("IfName", "none")
|
|
json.put("IfMTU", 65535)
|
|
|
|
if (json.getJSONArray("MulticastInterfaces").get(0) is String) {
|
|
var ar = JSONArray()
|
|
ar.put(0, JSONObject("""
|
|
{
|
|
"Regex": ".*",
|
|
"Beacon": true,
|
|
"Listen": true
|
|
}
|
|
""".trimIndent()))
|
|
json.put("MulticastInterfaces", ar)
|
|
}
|
|
}
|
|
}
|
|
|
|
fun getJSON(): JSONObject {
|
|
fix()
|
|
return json
|
|
}
|
|
|
|
fun getJSONByteArray(): ByteArray {
|
|
return json.toString().toByteArray(Charsets.UTF_8)
|
|
}
|
|
|
|
var multicastListen: Boolean
|
|
get() = (json.getJSONArray("MulticastInterfaces").get(0) as JSONObject).getBoolean("Listen")
|
|
set(value) {
|
|
updateJSON { json ->
|
|
(json.getJSONArray("MulticastInterfaces").get(0) as JSONObject).put("Listen", value)
|
|
}
|
|
}
|
|
|
|
var multicastBeacon: Boolean
|
|
get() = (json.getJSONArray("MulticastInterfaces").get(0) as JSONObject).getBoolean("Beacon")
|
|
set(value) {
|
|
updateJSON { json ->
|
|
(json.getJSONArray("MulticastInterfaces").get(0) as JSONObject).put("Beacon", value)
|
|
}
|
|
}
|
|
} |